gen-book
Generates a test book for manual testing. Also useful while developing a model, as a way of inspecting generated scenarios - even if the end goal is to generate automation-based tests.
Description
The gen-book
sub-command generates a manual test book based on a run source file, such as those created by the ensemble or sample sub-commands. Books can be generated either as static HTML websites, or as Excel files that can be imported to test execution management systems, such as HP QC/ALM.
During the book generation process, custom user code is invoked to translate test scenarios (i.e. event sequences) into a test scenarios. The book building user code uses a set of objects and methods developed specifically for this task - see full technical documentation below.
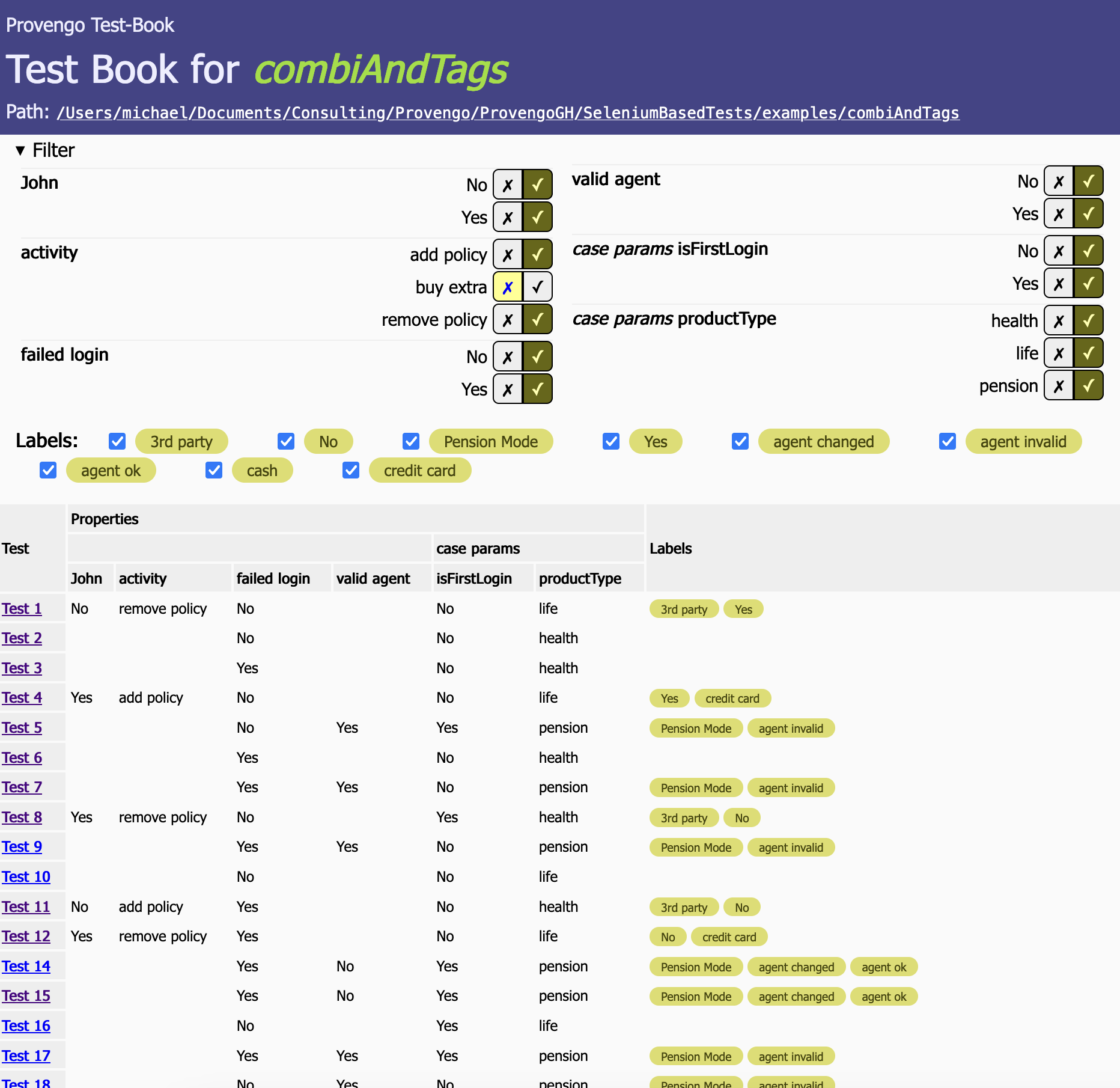
html
format.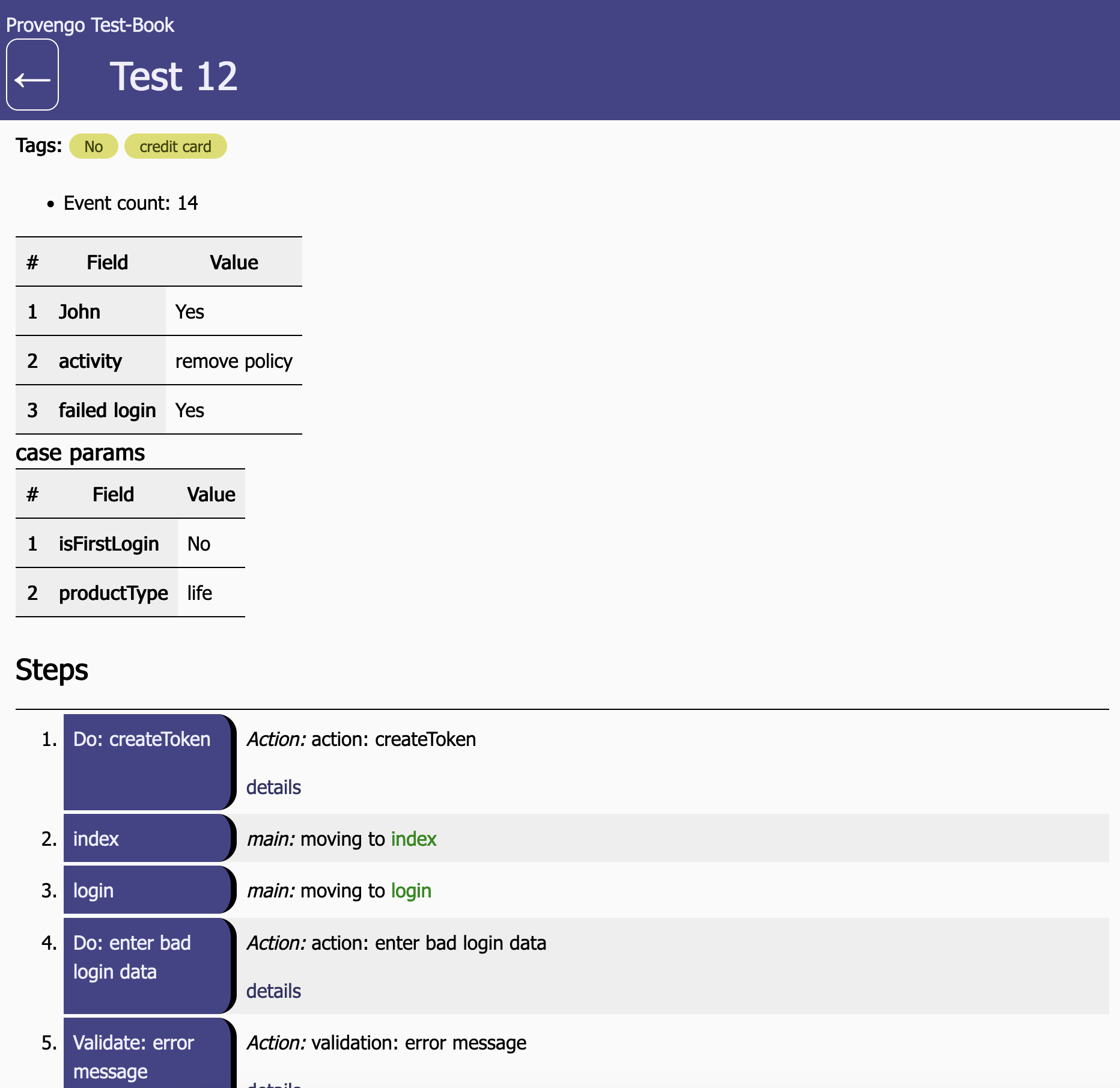
html
format.Command Parameters
-h
/--help
-
Display a help message and quit.
-f
/--format FORMAT_NAME
-
The format of generated test book. Defaults to
html
, which generates a test book in the form of a static web site. Another option isqc-xls
, which creates an Excel file ready to be imported into HP QC/ALM. --generator
/-g
TEST_BOOK_OBJECT-
Name of book generation object. Defaults to
TEST_BOOK
. -s
/--run-source TEST_BOOK_SOURCE
-
Path to a scenarios file to be used as source. Relative paths are resolved relatively to the project’s root directory. Defaults to
products/run-source/ensemble.json
. -o
/--output-file OUTPUT_FILE
-
Location for the generated test book.
API reference
Test book generation is performed by iterating the scenarios of a run-source file, and converting each of them to a manual test book scenario document. In the common case, each Event
in a scenario is translated into a single StepElement
in a manual test scenario. However, Event
s can also be ignored, or translated to more than a single step.
const TEST_BOOK = { (1)
documentEvent: function(event){ (2)
GenBook.autoTag(event); (3)
if ( event.data ){
TEST_SCENARIO.addElement( StepElement("Step", event.name) ); (4)
} else {
TEST_SCENARIO.addElement( StepElement("Step", event.name, event.data)); (5)
}
},
startTrace: function(){}, (6)
endTrace: function(){} (7)
};
1 | Defining the TEST_BOOK object. |
2 | The documentEvent function adds steps to the test scenario based on events it receives. |
3 | Automatically generate tags from certain event types. |
4 | Add a step to the manual test scenario being generated. |
5 | Same as above, but also remarks/expected result data to the step description. Note that we assume here that event.data is a primitive type, not an object or an array. |
6 | Empty start trace function |
7 | Empty end trace function. |
The API reference for objects involved in the creation of a manual test book it listed below.
A basic code for generating manual test books is automatically created for you when you use the create sub-command. It is available at ${project directory}/meta-spec/book-writer.js .
|
TEST_BOOK
This object is the entry point for the manual test book scenario generation code - the provengo
tool will look for it in the model. It will typically be stored in a JavaScript file in the meta-spec
directory, but it be in any JavaScript source directory. TEST_BOOK
is a regular JavaScript object, that contains the following three methods:
TEST_BOOK.startTrace()
-
Invoked by
provengo
when a trace is started. Does not take any parameters. Used mainly for initializing variables. TEST_BOOK.documentEvent(event)
-
Invoked by
provengo
for each of the scenario events (in order). Takes the event that needs to be documented as a parameter. TEST_BOOK.endTrace()
-
Invoked by
provengo
when a trace is completed. Does not take any parameters. Typically used for adding summary metadata to the generated manual testing scenario.
TEST_SCENARIO
The manual test scenario currently being built. Contains execution steps, header elements, and tags. It is initialized and stored automatically by the provengo
tool. Use the methods listed below to add content to a manual test.
TEST_SCENARIO.addMetadataLine(string)
-
Adds a metadata line to the scenario. This line will be shown at the top of the scenario page.
TEST_SCENARIO.addElement(emt)
-
Adds a
StepElement
to the test scenario body. TEST_SCENARIO.addHeaderElement(emt)
-
Same as above, but adds the element to the head part of the document.
TEST_SCENARIO.addTag(name, value)
-
Adds a named tag to the scenario. This tag can be used to filter scenarios at the test book index page.
name
can be aString
or anArray
ofString
s. TEST_SCENARIO.addTag(value)
-
Adds a value-only tag to the scenario. These tags are shown in the generated HTML as labels with round corners.
TEST_SCENARIO.setTitle(aTitle)
-
Sets the title of the scenario.
GenBook
Former helper object containing convenience methods useful for scenario building. Currently contains a single method, but we have a feeling this will change.
GenBook.autoTag(event)
-
Automatically generate tags for events created by
maybe()
,maybe(name)
,select(X).from(Y,Z,W)
,choice(X,Y,Z)
and any CombisetToEvent
. Returnstrue
if a tag was added, andfalse
otherwise.
ScenarioUtils
A helper object containing convenience methods useful for scenario building. Currently contains a single method, but we have a feeling this will change.
ScenarioUtils.autoTag(scenario)
-
Automatically generate tags for events created by
maybe()
,maybe(name)
,select(X).from(Y,Z,W)
,choice(X,Y,Z)
and any CombisetToEvent
. Returnstrue
if a tag was added, andfalse
otherwise. ScenarioUtils.autoTitle(scenario)
-
Automatically generate name for scenario, created by states names.
ScenarioUtils.addMetadata(scenario)
-
Automatically generate name and tags for scenario, using
autoTag
andautoTitle
functions
StepElement
A single step in a manual test scenario. Contains the step type, main text, and possible remarks.
When generating an Excel file for HP QC/ALM, the remarks field is used for storing the test step’s expected result.
|
StepElement(stepType, text, remarks)
Creates a new StepElement
object.
stepType
-
String. Type of step, e.g. "Validate", "Click". Appears on the left column of the page.
text
-
String. Main description of the step. Appears in the central column.
remarks
-
Optional String. When present, appears below
text
.
Example
The snippet below adds steps to the manual test scenario for each event that has a .data
field. For these, it takes the .data.lib
as the type of the step, the .data.action
as the main text, and the .data.details
as the remarks. For the type and text parameters, default values are provided where the .data
object does not provide a value.
function documentEvent( event ) {
if ( !!event.data ) {
let element = new StepElement(
(!!event.data.lib) ? event.data.lib : "Raw",
(!!event.data.action) ? event.data.action : event.name,
(!!event.data.details) ? event.data.details : null,
);
TEST_SCENARIO.addElement(element);
}
}
